148.排序链表
给你链表的头结点 head
,请将其按 升序 排列并返回 排序后的链表 。
示例 1:
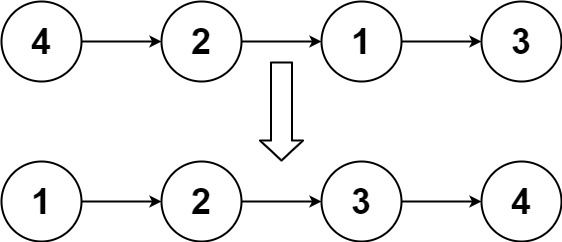
1 2
| 输入:head = [4,2,1,3] 输出:[1,2,3,4]
|
示例 2:
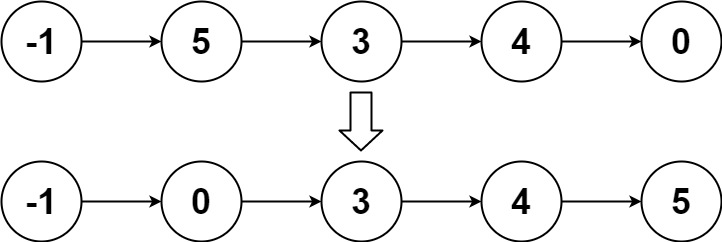
1 2
| 输入:head = [-1,5,3,4,0] 输出:[-1,0,3,4,5]
|
示例 3:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59
| class Solution { public ListNode sortList(ListNode head) { return mergeSort(head); }
ListNode mergeSort(ListNode head){ if(head == null || head.next == null){ return head; } ListNode mid = getMid(head); ListNode rightHead = mid.next; mid.next = null; ListNode leftSorted = mergeSort(head); ListNode rightSorted = mergeSort(rightHead); return mergeTwoLists(leftSorted, rightSorted); }
ListNode getMid(ListNode head){ ListNode slow = head, fast = head.next; while(fast != null && fast.next != null) { slow = slow.next; fast = fast.next.next; } return slow; }
ListNode mergeTwoLists(ListNode list1, ListNode list2) { ListNode dummy = new ListNode(); ListNode tail = dummy;
while(list1 != null && list2 != null) { if (list1.val <= list2.val) { tail.next = list1; list1 = list1.next; } else { tail.next = list2; list2 = list2.next; } tail = tail.next; }
tail.next = (list1 != null) ? list1 : list2; return dummy.next; } }
|