445.两数相加2
给你两个 非空 链表来代表两个非负整数。数字最高位位于链表开始位置。它们的每个节点只存储一位数字。将这两数相加会返回一个新的链表。
你可以假设除了数字 0 之外,这两个数字都不会以零开头。
示例1:
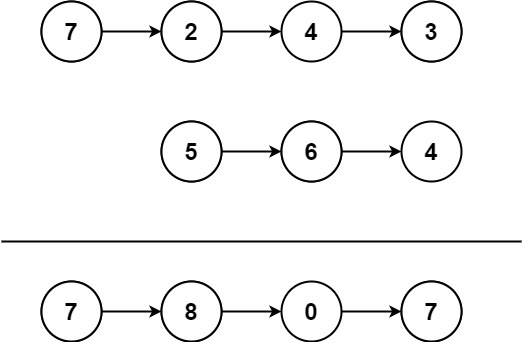
1 2
| 输入:l1 = [7,2,4,3], l2 = [5,6,4] 输出:[7,8,0,7]
|
示例2:
1 2
| 输入:l1 = [2,4,3], l2 = [5,6,4] 输出:[8,0,7]
|
示例3:
1 2
| 输入:l1 = [0], l2 = [0] 输出:[0]
|
Solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| class Solution {
public ListNode reverse1(ListNode l){ ListNode dummy =new ListNode(-1); dummy.next=l; ListNode pre=null; while(l!=null){ ListNode temp=l.next; l.next=pre; pre=l; l=temp; } return pre; } public ListNode addTwoNumbers(ListNode l1, ListNode l2) {
l1=reverse1(l1); l2=reverse1(l2); ListNode head = null, tail = null; int carry = 0;
while (l1 != null || l2 != null) { int n1 = l1 != null ? l1.val : 0; int n2 = l2 != null ? l2.val : 0; int sum = n1 + n2 + carry;
if (head == null) { head = tail = new ListNode(sum % 10); } else { tail.next = new ListNode(sum % 10); tail = tail.next; } carry = sum / 10;
if (l1 != null) { l1 = l1.next; } if (l2 != null) { l2 = l2.next; } } if (carry > 0) { tail.next = new ListNode(carry); } return reverse1(head); } }
|